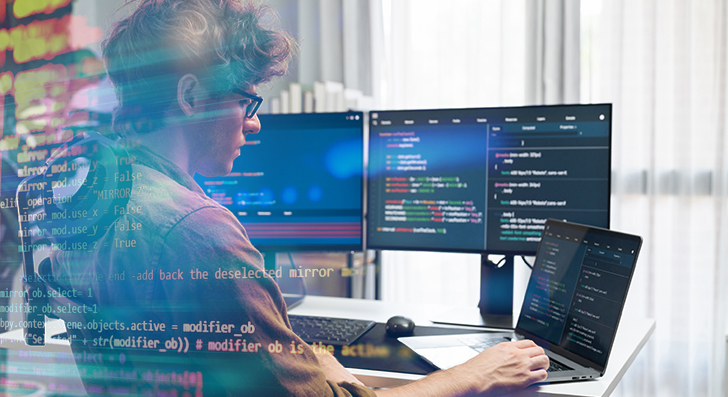
Scalability usually means your software can tackle expansion—far more customers, extra facts, plus much more site visitors—without having breaking. As a developer, making with scalability in mind will save time and pressure later on. Right here’s a transparent and functional guide to assist you to start off by Gustavo Woltmann.
Style for Scalability from the beginning
Scalability is not one thing you bolt on afterwards—it should be aspect of one's approach from the beginning. Lots of programs are unsuccessful after they expand speedy since the first design and style can’t tackle the extra load. For a developer, you have to Assume early about how your procedure will behave under pressure.
Start off by designing your architecture to get adaptable. Steer clear of monolithic codebases wherever every thing is tightly linked. As an alternative, use modular style and design or microservices. These patterns break your app into scaled-down, independent elements. Just about every module or service can scale By itself without the need of impacting the whole procedure.
Also, take into consideration your databases from working day 1. Will it want to deal with 1,000,000 people or simply just a hundred? Choose the appropriate style—relational or NoSQL—based on how your info will mature. Approach for sharding, indexing, and backups early, Even though you don’t will need them however.
One more vital stage is in order to avoid hardcoding assumptions. Don’t produce code that only is effective beneath latest disorders. Consider what would occur Should your consumer base doubled tomorrow. Would your app crash? Would the database slow down?
Use layout patterns that assistance scaling, like message queues or occasion-driven systems. These help your application tackle extra requests without receiving overloaded.
If you Construct with scalability in mind, you're not just preparing for fulfillment—you happen to be minimizing foreseeable future head aches. A very well-planned program is easier to take care of, adapt, and improve. It’s greater to organize early than to rebuild later.
Use the proper Databases
Choosing the ideal databases is actually a important part of making scalable apps. Not all databases are constructed the same, and utilizing the Mistaken one can gradual you down as well as trigger failures as your application grows.
Commence by comprehending your details. Could it be highly structured, like rows in a very table? If Sure, a relational databases like PostgreSQL or MySQL is a great match. These are solid with relationships, transactions, and regularity. They also guidance scaling strategies like browse replicas, indexing, and partitioning to take care of a lot more traffic and knowledge.
If your knowledge is more versatile—like person activity logs, product or service catalogs, or documents—consider a NoSQL selection like MongoDB, Cassandra, or DynamoDB. NoSQL databases are better at dealing with significant volumes of unstructured or semi-structured info and will scale horizontally much more simply.
Also, consider your go through and generate designs. Are you carrying out many reads with fewer writes? Use caching and browse replicas. Will you be handling a large produce load? Take a look at databases that will deal with substantial generate throughput, or even occasion-dependent details storage systems like Apache Kafka (for short-term knowledge streams).
It’s also clever to Imagine ahead. You might not need Sophisticated scaling functions now, but picking a databases that supports them suggests you received’t have to have to modify afterwards.
Use indexing to speed up queries. Keep away from avoidable joins. Normalize or denormalize your data according to your entry styles. And normally observe databases performance as you grow.
In short, the best databases will depend on your application’s framework, pace wants, And the way you count on it to expand. Get time to choose wisely—it’ll preserve a great deal of difficulties later on.
Enhance Code and Queries
Quickly code is key to scalability. As your application grows, each individual smaller hold off adds up. Poorly penned code or unoptimized queries can slow down overall performance and overload your system. That’s why it’s important to Establish successful logic from the start.
Begin by writing clean up, uncomplicated code. Keep away from repeating logic and remove everything needless. Don’t choose the most elaborate Resolution if a simple a person will work. Maintain your capabilities limited, targeted, and straightforward to test. Use profiling resources to uncover bottlenecks—destinations exactly where your code takes far too extended to operate or employs an excessive amount of memory.
Future, have a look at your databases queries. These typically sluggish matters down a lot more than the code itself. Be sure Every question only asks for the data you really have to have. Keep away from SELECT *, which fetches anything, and rather choose precise fields. Use indexes to speed up lookups. And keep away from doing too many joins, Primarily across massive tables.
If you recognize a similar information staying requested time and again, use caching. Store the outcomes briefly applying resources like Redis or Memcached therefore you don’t have to repeat pricey functions.
Also, batch your databases functions whenever you can. As opposed to updating a row one by one, update them in groups. This cuts down on overhead and can make your application more effective.
Remember to examination with substantial datasets. Code and queries that do the job good with 100 information may possibly crash every time they have to deal with 1 million.
In a nutshell, scalable applications are rapid applications. Keep the code limited, your queries lean, and use caching when needed. These actions assist your application remain easy and responsive, even as the load boosts.
Leverage Load Balancing and Caching
As your application grows, it's got to manage additional buyers and more visitors. If every thing goes by means of a single server, it's going to speedily become a bottleneck. That’s in which load balancing and caching are available in. These two resources enable keep the application rapid, secure, and scalable.
Load balancing spreads incoming targeted visitors throughout many servers. In lieu of a person server accomplishing many of the perform, the load balancer routes consumers to unique servers determined by availability. This implies no single server gets overloaded. If one server goes down, the load balancer can mail visitors to the Other individuals. Resources like Nginx, HAProxy, or cloud-centered alternatives from AWS and Google Cloud make this very easy to setup.
Caching is about storing data quickly so it could be reused swiftly. When customers ask for precisely the same info all over again—like an item web page or simply a profile—you don’t ought to fetch it in the databases each and every time. You are able to provide it from your cache.
There are two popular varieties of caching:
one. Server-aspect caching (like Redis or Memcached) stores knowledge in memory for quick entry.
2. Customer-side caching (like browser caching or CDN caching) merchants static files near to the person.
Caching decreases database load, increases speed, and would make your app far more efficient.
Use caching for things that don’t improve usually. And normally ensure your cache is current when info does improve.
In short, load balancing and caching are basic but effective applications. With each other, they assist your application deal with additional users, remain rapid, and recover from difficulties. If you intend to grow, you will need both equally.
Use Cloud and Container Tools
To construct scalable apps, you'll need equipment that permit your application grow very easily. That’s the place cloud platforms and containers are available. They offer you flexibility, decrease setup time, and make scaling Substantially smoother.
Cloud platforms like Amazon Website Services (AWS), Google Cloud Platform (GCP), and Microsoft Azure Allow you to rent servers and products and services as you need them. You don’t must get components or guess long run ability. When targeted visitors increases, you are able to include a lot more methods with just a couple clicks or mechanically applying vehicle-scaling. When targeted visitors drops, you could scale down to save cash.
These platforms also supply providers like managed databases, storage, load balancing, and safety equipment. It is possible to target constructing your app as opposed to handling infrastructure.
Containers are An additional important tool. A container offers your application and almost everything it must operate—code, libraries, configurations—into a person device. This causes it to be simple to move your application among environments, out of your notebook to your cloud, with no surprises. Docker is the most well-liked tool for this.
Once your app utilizes various containers, instruments like Kubernetes allow you to handle them. Kubernetes handles deployment, more info scaling, and Restoration. If one aspect of the app crashes, it restarts it mechanically.
Containers also ensure it is easy to individual elements of your application into providers. You can update or scale pieces independently, which can be perfect for effectiveness and reliability.
To put it briefly, making use of cloud and container tools signifies you are able to scale fast, deploy quickly, and recover promptly when issues transpire. If you would like your application to grow with no restrictions, commence applying these resources early. They help save time, decrease chance, and help you remain centered on building, not repairing.
Watch Almost everything
If you don’t check your software, you received’t know when issues go Improper. Checking allows you see how your app is doing, location issues early, and make far better selections as your application grows. It’s a vital Element of developing scalable programs.
Start out by monitoring simple metrics like CPU utilization, memory, disk Place, and reaction time. These tell you how your servers and solutions are carrying out. Instruments like Prometheus, Grafana, Datadog, or New Relic will let you obtain and visualize this data.
Don’t just keep track of your servers—check your app also. Control just how long it will require for people to load internet pages, how frequently glitches transpire, and where by they manifest. Logging applications like ELK Stack (Elasticsearch, Logstash, Kibana) or Loggly will help you see what’s taking place inside your code.
Setup alerts for essential issues. For instance, Should your response time goes previously mentioned a limit or perhaps a services goes down, you need to get notified instantly. This helps you fix challenges speedy, generally in advance of end users even recognize.
Monitoring is also practical whenever you make changes. For those who deploy a different attribute and see a spike in faults or slowdowns, you may roll it back again just before it causes serious hurt.
As your app grows, targeted visitors and facts boost. Without checking, you’ll skip indications of difficulties till it’s much too late. But with the best resources set up, you remain on top of things.
Briefly, monitoring allows you maintain your application reputable and scalable. It’s not just about recognizing failures—it’s about understanding your process and ensuring it really works nicely, even stressed.
Final Feelings
Scalability isn’t just for huge companies. Even modest applications want a solid foundation. By developing diligently, optimizing properly, and utilizing the right equipment, you could Construct apps that increase effortlessly without having breaking stressed. Start tiny, Assume big, and Create good.